The First Draft
I've written my fair share of web pages over the years—eighteen years or so. But if I prompt an AI to write one for me, it delivers a far better markup—faster, committed to the golden rules of semantics, and always mindful of accessibility. That very first draft of markup that AI churns out? It's beautiful—no denying it.
In the real world, we take that AI markup—typically written in traditional HTML, BEM, or Tailwind—and iterate on it… excessively. Here’s the thing: the more we prompt models to refine their own code, the more likely it is that the iterated output loses semantic integrity and pattern consistency.
We don't need another framework to fix this issue; we need a methodology that understands the underlying issues. Our traditional HTML crumbles when scaled aggressively, BEM drowns us in verbosity, and Tailwind strips the semantic layer; none of these were built with AI-driving iterations in mind—and it shows in our increasingly expensive development cycles.
The Challenge
Transformer models convert our code into vector embeddings—mathematical representations capturing meaning in high-dimensional space. More importantly, these models thrive on pattern recognition and sequence-based tasks. Our current methodologies don’t leverage these strengths. Instead, they push models to process these deep hierarchies, parse verbose naming conventions, or (attempt to) maintain semantic relationships through nameless utility classes. With each iteration, the pattern consistency degrades in the process—because:
- Our original components mutate into deep, unanchored hierarchies.
- Clear relationships blur into ambiguous tokens, eventually kicked out of the context window.
- Semantic relationships fragment across multiple naming patterns, making it difficult for models to maintain consistent meaning.
Developer custody of code becomes difficult.
Models don't necessarily use diff checks; they rely on their mental modal made of the context window with a few fancy memory techniques.
Think about it: with each iteration, we're asking models to infer relationships and maintain patterns through their context window. Traditional approaches make this harder than it needs to be.
- As components evolve, each new class or state can significantly inflate our token count, driving up computational overhead and context window usage.
- Base patterns mutate through successive modifications, making it harder for models to recognize and maintain original semantic relationships.
- Features lose their context because there's no stable reference point between iterations, breaking recently established relationships in favor of new ones.
- Refactoring becomes increasingly tricky as semantic boundaries blur with each modification.
Methodology, Not a Framework
Blocktail uses semantic markers that maintain stability through iterations...
But it’s not just about these markers—Blocktail’s advantage is in how it systematically reduces repetition, preserves token boundaries, and keeps the token count low so that features evolve predictably.
- Component references that provide clear anchors for features to evolve predictably.
- State management that both humans and machines can parse and debug easily.
- Patterns that add features through predictive evolution rather than deconstruction.
- Structure that keeps semantic relationships intact across iterations.
- Developers can quickly reclaim code custody.
Central to this methodology is how it interacts with AI-driven workflows, particularly in optimizing token economy and preserving context over iterations.
The Token Economy
- Reduced Repetition & Improved Token Vocabulary
- By referencing blocks once and layering on concise tails or mutations, we minimize duplicated fragments. AI sees a smaller, more stable subword vocabulary that is less prone to pattern drifts.
- Consistent Token Boundaries & Fewer Subwords
- Each class is just a few tokens, making the naming predictable. Short, stable classes reduce subword fragmentation and chain-of-thought decay.
- Token Economy & Context Window
- More concise class names free up tokens in the model’s context. This means deeper logic can stay in play over repeated AI-driven code refinements.
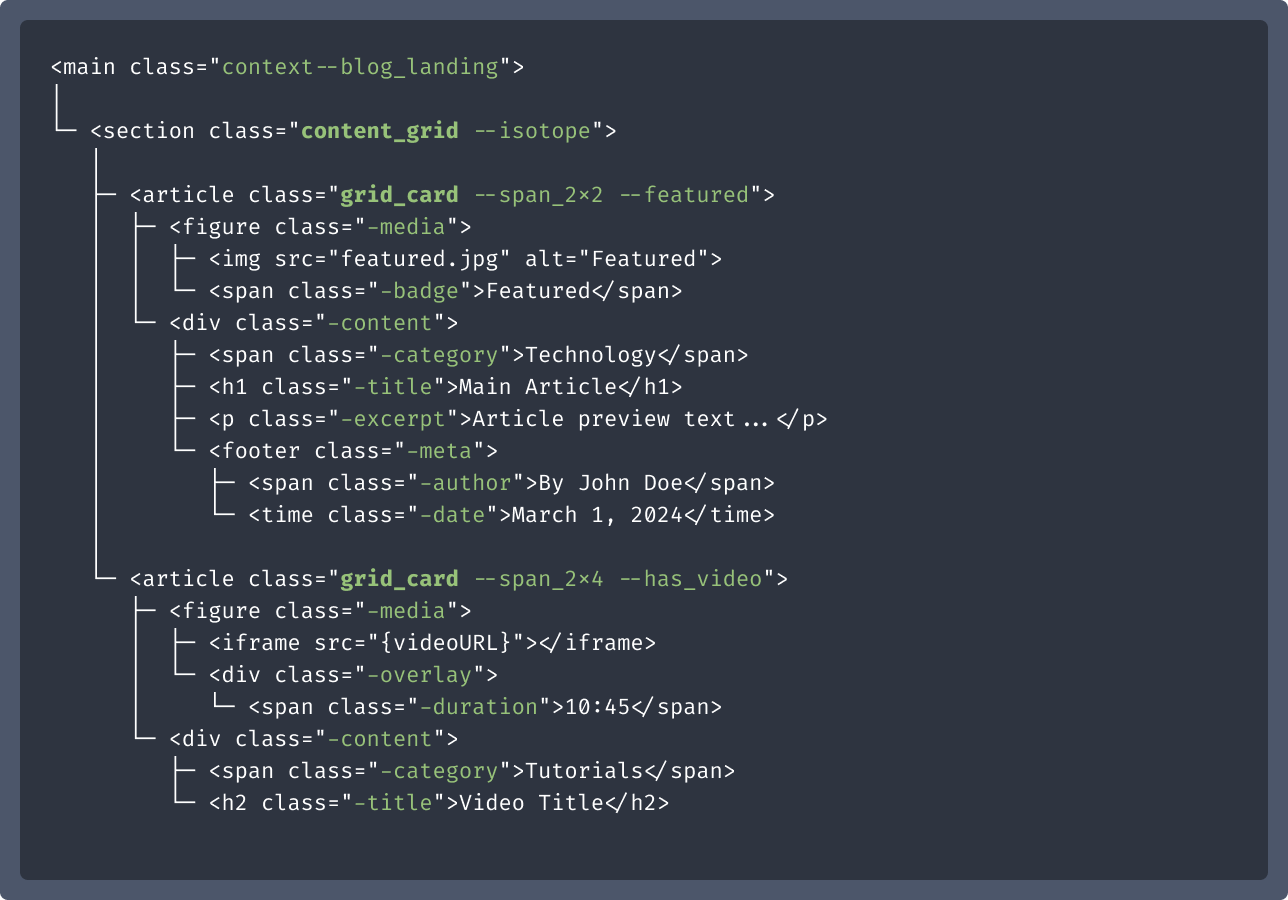
Pre-trained models primarily operate in inference mode during practical use, applying learned patterns to new inputs without modifying their core understanding. While these models can understand and work with various coding patterns, consistent naming conventions and structural relationships like those in Blocktail can help produce more maintainable and predictable code.
The Layer System
When we talk about maintaining pattern stability, it all comes down to boundaries. Think of these boundaries like abstraction layers in software architecture—each serving a distinct purpose and isolating structural complexities. Blocktail organizes these boundaries into five distinct layers, each serving as a clear marker for both machines and humans:
<body class="matrix--shop"> <!-- L1: System Context -->
<main class="context--catalog"> <!-- L2: Local Context -->
<article class="product_card <!-- L3: Component -->
--featured <!-- L4: Mutation -->
data-observer="ProductObserver">
<h2 class="-title"> <!-- L5: Element -->
Product Name
</h2>
</article>
</main>
</body>
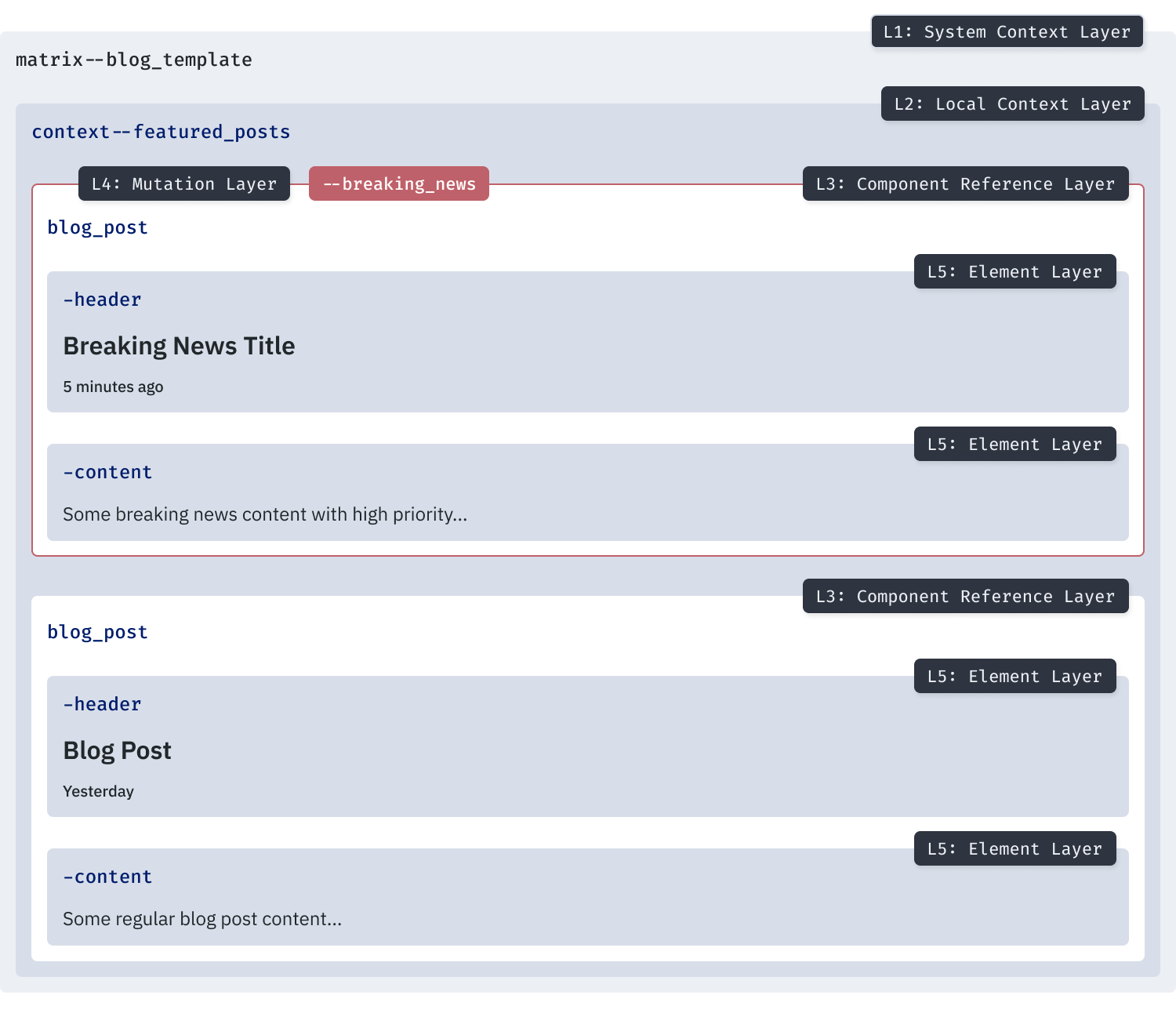
L1: System Context Layer
The System Context Layer, marked by matrix--
, is an optional system-level marker (only if you have multiple base templates). It represents the overarching structure or template layout for a web page.
<body class="matrix--shop">
<!-- "matrix--shop": Marks this page as part of the e-commerce system -->
</body>
L2: Local Context Layer
The Local Context Layer, marked by context--
, is a fundamental concept in Blocktail that provides stable realities or themes for our blocks. Rooted in psychological principles, context offers a powerful way to create adaptive, maintainable, and intuitive page structures.
<main class="context--catalog">
<!-- "context--catalog": Defines a thematic scope for the product listing -->
<section class="context--seasonal">
<!-- Seasonal promotions adapt to the catalog theme -->
<article class="product_card --featured">...</article>
</section>
<section class="context--best_sellers">
<!-- Best-selling products inherit catalog styling while adding a unique theme -->
<article class="product_card">...</article>
</section>
</main>
L3: Component Reference Layer
The Component Reference Layer is where blocks live. A block in Blocktail is a self-contained, reusable component that represents a distinct section or element of a web page. Blocks are the primary building units of our interface:
<article class="product_card --featured">
<!-- "product_card": Self-contained block for a product -->
<!-- "--featured": Adds emphasis without altering the block's base behavior -->
<h2 class="-title">Popular Product</h2>
<p class="-description">Brief product details go here.</p>
<span class="-price">$49.99</span>
</article>
L4: Mutation Layer
Mutations work like command-line flags, providing an additive approach to state management—you can stack them, remove them, or modify them without disrupting the block's core behavior. Just as command-line flags let you toggle features without rewriting the program, mutations let you scale functionality up or down on demand:
<article class="product_card --on_sale --limited_stock">
<!-- "product_card": Base block remains untouched -->
<!-- "--on_sale": Signals the item is discounted -->
<!-- "--limited_stock": Highlights availability constraints -->
<h2 class="-title">Flash Sale Item</h2>
<span class="-price --discounted">$24.99</span>
</article>
L5: Element Layer
The Element Layer, marked by -
, defines tails. Each block is made of tails. A tail provides a way to structure and style elements within a block without adding verbosity. It represents a substructure that remains scoped to its parent block.
<nav class="main_navigation">
<!-- "main_navigation": Self-contained navigation block -->
<ul class="-menu">
<!-- "-menu": Scoped tail representing the navigation list -->
<li class="-item"><a href="#" class="-link">Home</a></li>
<li class="-item"><a href="#" class="-link">Shop</a></li>
</ul>
</nav>
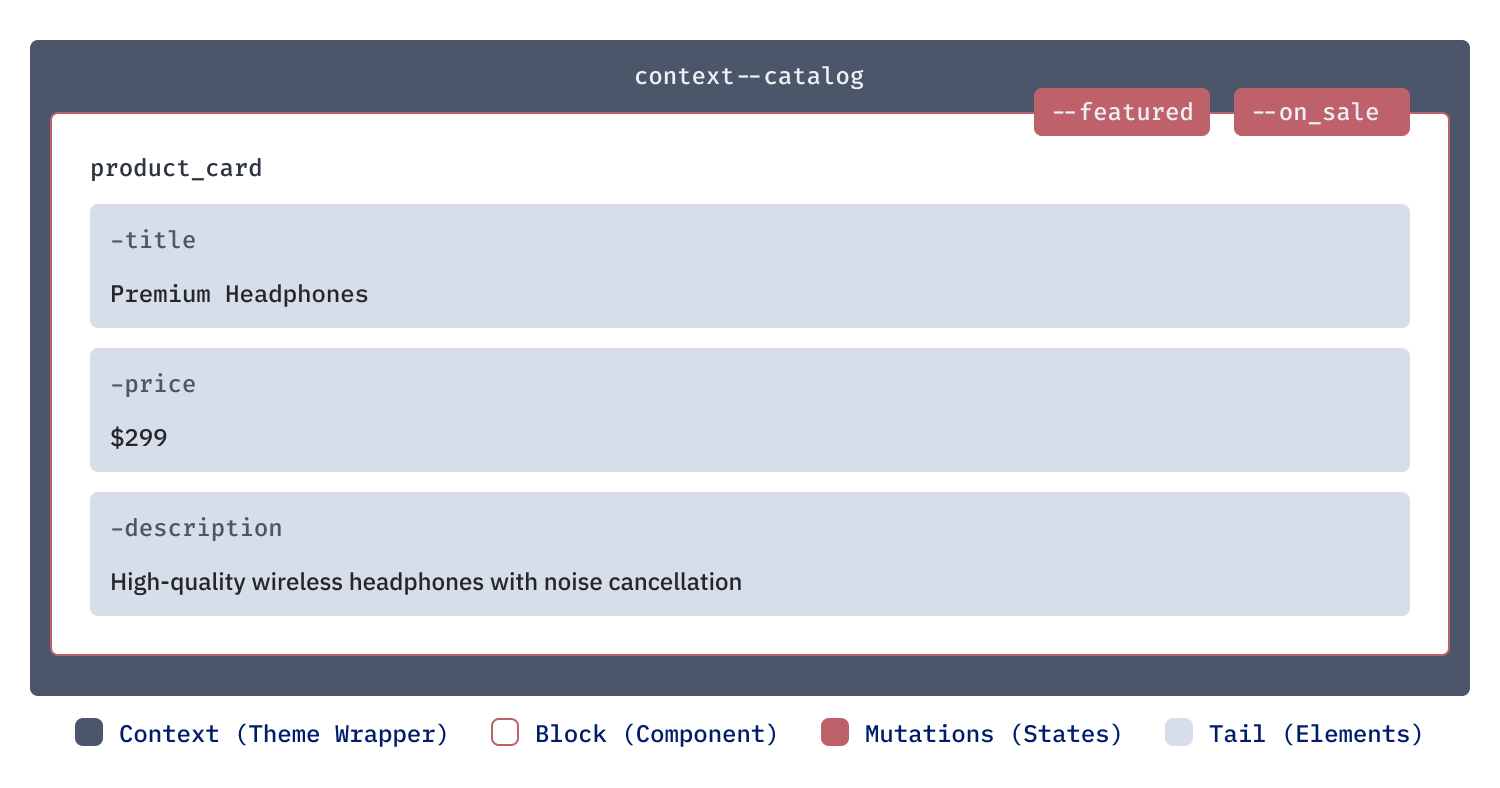
Under The Layers
Blocktail is a block-based component architecture that frames design patterns as modular, reusable units. It's not a library but a framework-agnostic methodology that seamlessly integrates into your workflow, requiring no tools or dependencies.
It uses purpose-driven pattern syntax and is ideal for large distributed teams, mission-critical web apps, and AI-driven dev pipelines.
Read more at Blocktail.dev